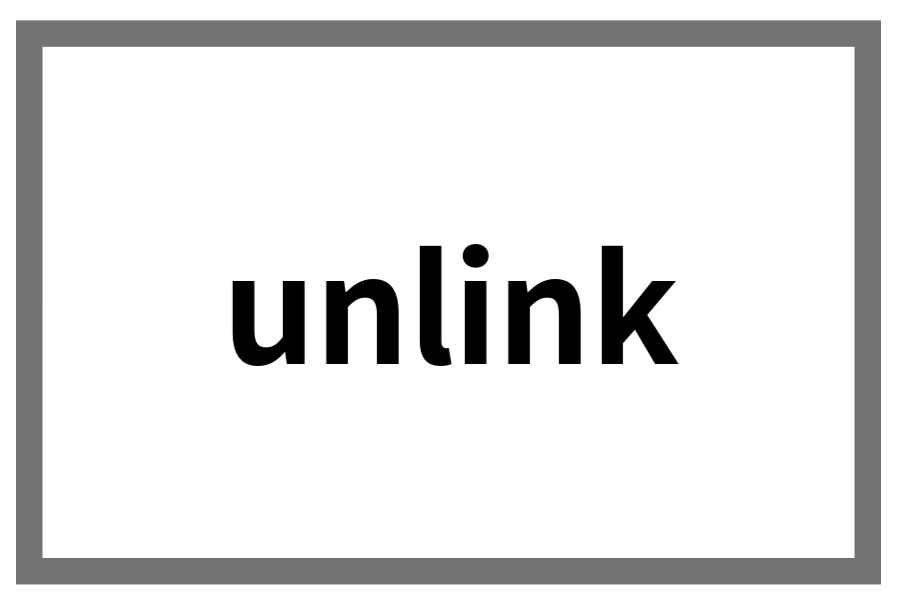
📌 매뉴얼 (Linux)
NAME
unlink - delete a name and possibly the file it refers to
: 이름과 파일을 삭제
SYNOPSIS
#include <unistd.h>
int unlink(const char *pathname);
DESCRIPTION
unlink() deletes a name from the filesystem. If that name was
the last link to a file and no processes have the file open, the
file is deleted and the space it was using is made available for
reuse.
If the name was the last link to a file but any processes still
have the file open, the file will remain in existence until the
last file descriptor referring to it is closed.
If the name referred to a symbolic link, the link is removed.
If the name referred to a socket, FIFO, or device, the name for
it is removed but processes which have the object open may
continue to use it.
: undlink()는 파일 시스템에서 이름을 삭제합니다. 해당 이름이 파일에 대한 마지막 링크이고
파일이 열리지 않는 프로세스가 없는 경우, 파일은 삭제되고 파일이 사용 중이던 공간이 재사용 가능하게 됩니다.
이름이 파일에 대한 마지막 링크였지만 프로세스에 파일이 열려 있는 경우 해당 파일을 참조하는
마지막 파일 설명자가 닫힐 때까지 파일은 그대로 유지됩니다.
이름이 기호 링크를 참조하면 링크가 제거됩니다.
이름이 소켓, FIFO 또는 장치를 언급한 경우 해당 소켓의 이름은 제거되지만
개체가 열려 있는 프로세스는 계속해서 이 소켓을 사용할 수 있습니다.
RETURN VALUE
On success, zero is returned. On error, -1 is returned, and
errno is set to indicate the error.
: 성공 시 0이 반환되며, 오류 시 -1이 반환되고 errno가 설정되어 오류를 나타냅니다.
📌 함수 설명
unlink 함수는 파일을 삭제하는 system call 함수로서, hard link의 이름을 삭제하고
hard link가 참조하는 count를 1 감소시킨다.
여기서 hard link 란, '원본 파일과 동일한 i-node (파일의 고유 번호, 파일 생성시 주어짐.) 를 가지는 복사본' 으로,
cp로 만드는 일반 복사본과 다르게, 원본을 수정하든 hard link 복사본을 수정하든 함께 수정된다.
i-node 로 1234를 가지고 있는 파일이 수정되면 같은 i-node : 1234를 가진 모든 파일들이 같이 수정되는 것이다.
따라서, 원본 파일이 지워져도 hard link 파일은 보존되어 실행된다.
(반대되는 개념으로 soft link는 i-node가 달라 원본이 지워지면 링크 연결이 끊기게 된다.)
그리하여, 위에서 언급한 unlink를 통해 hard link가 참조하는 count가 줄어들어 0이 되면,
원본과 hard link 모두 지워진 상태이므로, 해당 파일의 내용이 저장된 disk 공간을 free하게 된다.
hard link가 없는 일반 파일은 바로 disk 공간을 free하여 사실상 파일을 삭제하는 것이다.
함수의 인자로는 const char* pathname 을 받는데, 이는 삭제할 파일명이며 (full path 또는 상대 path)
return 값으로는 '정상적으로 file 또는 link가 삭제'되면 0을 반환하고,
'오류가 발생'하면 -1을 반환하고 오류내용이 errno에 저장된다.
#include <unistd.h>
int main()
{
unlink("testabc");
return (0);
}
실행 결과)
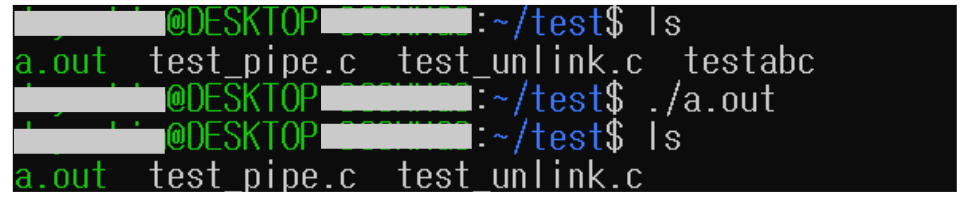
단순히 testabc라는 파일을 unlink 하여 삭제해주는 코드이다. 실행 시, 해당 파일이 삭제된 모습을 볼 수 있다.
해당 파일의 hard link가 존재하지 않으므로 바로 disk 공간을 free해준 상태이다.
특수한 상황이 있는데, 지우려는 파일이 더 이상 연결된 hard link가 없지만, open되어 있는 경우에는
파일이 close될 때까지 파일을 remain, 유지 시켜준다.
#include <sys/types.h>
#include <sys/stat.h>
#include <unistd.h>
#include <fcntl.h>
#include <stdio.h>
int main()
{
int fd = 0;
fd = open("test_unlink", O_WRONLY | O_CREAT | O_TRUNC);
unlink("test_unlink");
char buf[10] = "good";
int word_len = write(fd, buf, 10);
if (word_len == 10)
printf("write O\n");
else
printf("write X\n");
close(fd);
return (0);
}
결과 - std_output)
write O |
open을 하고, 바로 unlink한 상황인데, 해당 파일이 close가 되기 전까지는 파일을 유지시켜주는 특징이 있다.
따라서 이후에 write를 하면, 정상적으로 write가 되는 모습을 볼 수 있다.
그리고, close를 통해 마지막에 닫고 해당 파일은 지워지게 된다.
'프로그래밍 > C' 카테고리의 다른 글
[function] waitpid 함수 알아보기 (2) | 2024.06.13 |
---|---|
[function] wait 함수 알아보기 (0) | 2024.06.09 |
[function] pipe 함수 알아보기 (0) | 2024.06.06 |
[function] fork 함수 알아보기 (2) | 2024.06.04 |
[function] exit 함수 알아보기 (0) | 2024.05.31 |